SwiftUI’s ability to quickly create UI is very powerful, and Apple also provides a PDFKit framework, so you can use SwiftUI and PDFKit to create a fully customizable PDF reader with very little code, very quickly.
Let’s start from scratch and make a PDF reader.
Create a project
First, open Xcode, and create a new iOS App. The project name can be PdfReader. Interface select Swift UI, language select Swift.
Because PDFKit is a UIKit library, not the native SwiftUI object, we first need to make a class to bridge the two. I’ve already prepared one for you. You need to create a SwiftPDFView.swift and copy the following code.
//
// SwiftPDFView.swift
// PdfReader
//
// Created by HaoPeiqiang on 2023/1/3.
//
import SwiftUI
import PDFKit
struct SwiftPDFView: UIViewRepresentable {
let url:URL
func makeUIView(context: Context) -> PDFView {
let pdfView = PDFView()
pdfView.document = PDFDocument(url: url)
pdfView.displayMode = .singlePage
pdfView.autoScales = true
pdfView.usePageViewController(true, withViewOptions: nil)
return pdfView
}
func updateUIView(_ pdfView: PDFView, context: Context) {
}
}
struct SwiftPDFView_Previews: PreviewProvider {
static var previews: some View {
SwiftPDFView(url:Bundle.main.url(forResource: "sample_pdf", withExtension: "pdf")!)
}
}
Then you can find the automatic generation of ContentView.swift file, remove the automatic generation code inside the body, add SwiftPDFView(url: Bundle.main.url(forResource: "sample_pdf", withExtension: "pdf")!)
. Well, you have successfully created a PDF reader in less than three minutes.
Suppose you open the Xcode preview area on the right side of the code area. You do not have to run the App. You can already see that your PDF viewer has been able to work, and it can also navigate through the different PDF pages.
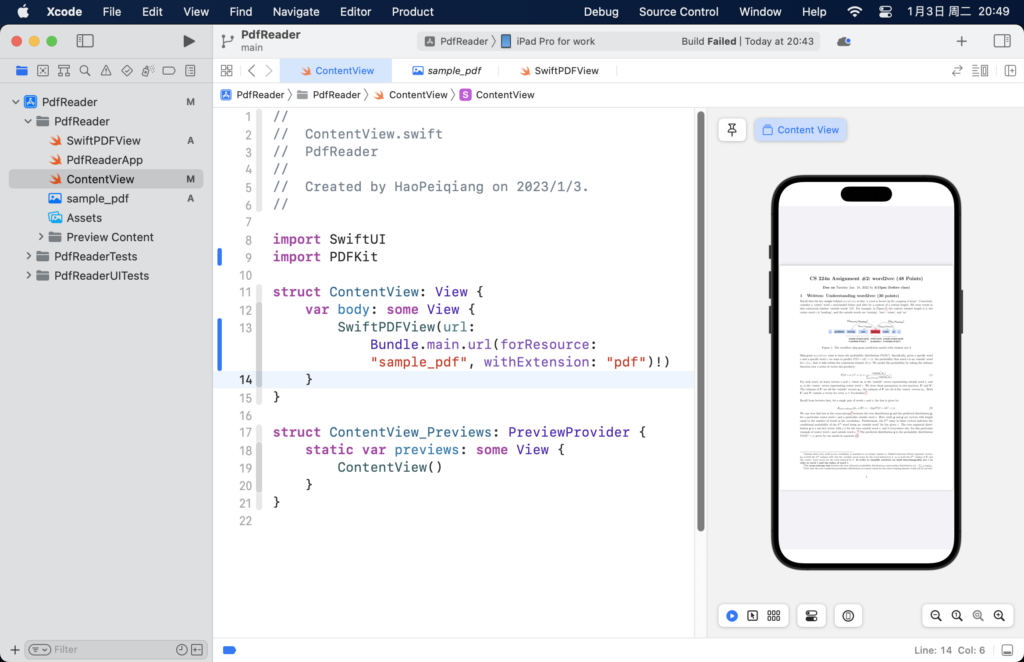
Add thumbnail navigation bar
You may have seen some PDF App, there is a row of thumbnails at the bottom of the PDF page, and you can navigate and also preview the contents of the PDF file, which is called PDFThumbnailView. join PDFThumbnailView can make our PDF reader look more professional.
We can return to the SwiftPDFView.swift file in the SwiftPDFView class code added to the following code inside.
func setThumbnailView(_ pdfView:PDFView) {
let thumbnailView = PDFThumbnailView()
thumbnailView.translatesAutoresizingMaskIntoConstraints = false
pdfView.addSubview(thumbnailView)
thumbnailView.leadingAnchor.constraint(equalTo: pdfView.safeAreaLayoutGuide.leadingAnchor).isActive = true
thumbnailView.trailingAnchor.constraint(equalTo: pdfView.safeAreaLayoutGuide.trailingAnchor).isActive = true
thumbnailView.bottomAnchor.constraint(equalTo: pdfView.safeAreaLayoutGuide.bottomAnchor).isActive = true
thumbnailView.bottomAnchor.constraint(equalTo: pdfView.safeAreaLayoutGuide.bottomAnchor).isActive = true
thumbnailView.heightAnchor.constraint(equalToConstant: 40).isActive = true
thumbnailView.thumbnailSize = CGSize(width: 20, height: 30)
thumbnailView.layoutMode = .horizontal
thumbnailView.pdfView = pdfView
}
Then at the makeUIView
method, before return pdfView
adding a self.setThumbnailView (pdfView)
.
Then, view the preview area, you will find that the thumbnail navigation bar below the PDF display area.
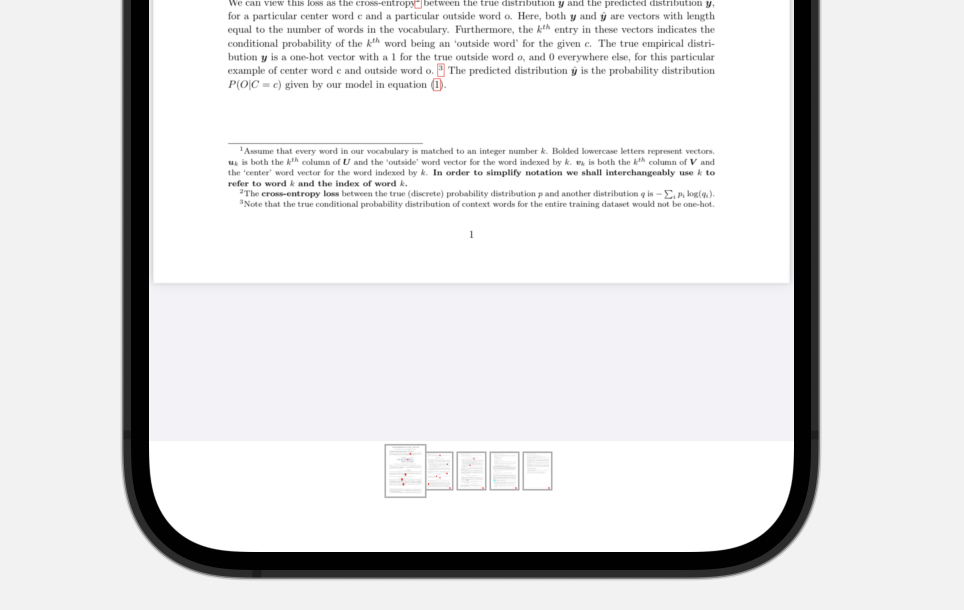
The project code can be downloaded from GitHub https://github.com/Swift-Cast/PdfReader.